Extending / Intermediate / Back-end Menu Items
Note: You are currently reading the documentation for Bolt 3.7. Looking for the documentation for Bolt 5.2 instead?
Bolt allows extensions to insert items below the Extensions
menu in the admin
UI.
Bolt provides a helper function that will allow additional MenuEntry
objects
to be added to the admin UI menus when they are built in the
Bolt\Menu\AdminMenuBuilder
class.
Registering Menu Entries¶
Menu objects for registration can be created using a Bolt\Menu\MenuEntry
class object.
protected function registerMenuEntries()
{
$menu = MenuEntry::create('koala-menu', 'koala')
->setLabel('Koala Catcher')
->setIcon('fa:leaf')
->setPermission('settings')
->setRoute('KoalaExtension')
;
return [
$menu,
];
}
In the above example:
koala-menu
parameter in the constructor is used internally and should just be a brief name, and only needs to be unique for submenus of Extend.koala
parameter in the constructor will set the route for the menu to bebolt/extend/koala
(where branding path is still set tobolt/
setLabel('Koala Catcher')
sets the displayed menu label in the left side bar to "Koala Catcher"setIcon('fa:leaf')
set the icon for the menu to any of the Font Awesome iconssetPermission('settings')
sets the required permission, as defined in theapp/config/permissions.yml
filesetroute('KoalaExtension')
sets the route to "KoalaExtension", which is used for generating paths and URLs, like in{{ path() }}
. Strictly speaking this is optional, but we strongly recommend it, to prevent problems with the routing in non-trivial applications.
Note: Menu entries are mounted on extensions/, because they fall under Extensions, logically. When adding an accompanying route for a new menu item, make sure to catch it correctly. For the above example, it should match /extensions/koala.
Adding submenus¶
Similarly as described above, you can add submenu items to a menu, by "adding" them to a previously defined menu item. Below is a full example of how to add a menu with two submenu items:
protected function registerMenuEntries()
{
$menu = MenuEntry::create('koala-menu', 'koala')
->setLabel('Koala Catcher')
->setIcon('fa:leaf')
->setPermission('settings')
;
$submenuItemOne = MenuEntry::create('koala-submenu-one', 'koala-tree')
->setLabel('Koala One')
->setIcon('fa:tree')
;
$submenuItemTwo = MenuEntry::create('koala-submenu-two', 'koala-food')
->setLabel('Koala Two')
->setIcon('fa:tree')
;
$menu->add($submenuItemOne);
$menu->add($submenuItemTwo);
return [
$menu,
];
}
The result will look like this:
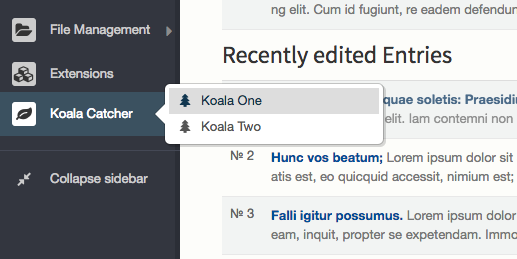
Note: For the sake of brevity, we omitted ->setRoute() and ->setPermission() in the above example. In your own code, you should add these.
Couldn't find what you were looking for? We are happy to help you in the forum, on Slack or on Github.